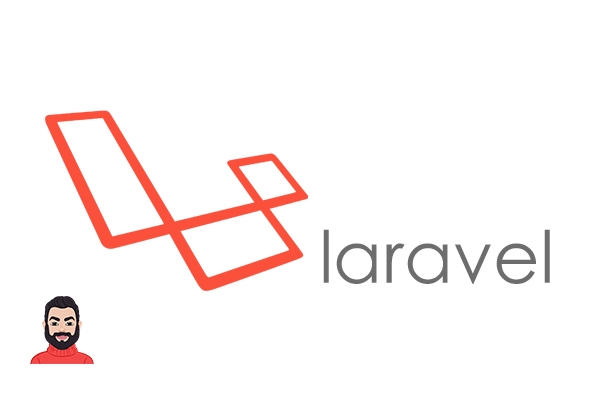
Here you will learn how to remove the last element from a collection in laravel. This article will give you a simple example of the laravel collection's last item being removed. This article will give you a simple example of laravel removing the last item from the collection.
You have just to follow the below step and you will get the layout as below:
- Setup Laravel
composer create-project laravel/laravel example-app
- Assign a Route in Web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\CollectionRemoveLastController;
Route::get('/index',[CollectionRemoveLastController::class, 'index']);
- Create Controller by enter this in terminal
CollectionRemoveLastController
& now go in that controller to input the code below.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class CollectionRemoveLastController extends Controller
{
/**
* The attributes that are mass assignable.
*
* @var array
*/
public function index()
{
$Collection = collect(['x','y','z']);
$newCollection = $Collection->reverse()->slice(1)->reverse();
dd($newCollection);
}
}
- Now run your project with this command
php artisan serve
and you will get the result once you are in that route we defined previously.
#items: array:2 [▼
0 => "x"
1 => "y"
]
You can use this example with the versions of laravel 6, laravel 7, laravel 8, and laravel 9.
Read my others post
Dont forget to comment if need any help
← Previous postForm Request Has File Check in Laravel